Object-Oriented Programming with Java: From Basics to Advanced Concepts
What Will You Learn?
In this Java Programming course, you’ll begin by building a strong foundation in Java syntax and structure, which will enable you to write clean and efficient code. You’ll gain a comprehensive understanding of data types and operators, learning how to use decision-making constructs like if-else and switch-case to control the flow of your programs. The course also covers arrays, where you’ll learn how to create, manipulate, and manage arrays for efficient data handling, providing a strong base for more advanced topics.
As you advance, you’ll explore Object-Oriented Programming (OOP) principles such as encapsulation, inheritance, and polymorphism, which are key to designing robust, scalable applications. You’ll also master exception handling using try-catch blocks to ensure your applications are more reliable and can manage errors gracefully. Finally, you’ll discover how to implement multithreading, enabling the creation and management of multiple threads for concurrent task execution, which enhances your programs’ performance and efficiency.
Course Flow
The Java Programming course begins with an introduction to Java basics, where you’ll learn the syntax, structure, and essential concepts of the language. You’ll explore data types, operators, and decision-making constructs to control program flow. The course then progresses to arrays, teaching you how to efficiently manage data, followed by an in-depth look at Object-Oriented Programming (OOP) principles like encapsulation, inheritance, and polymorphism for robust application design.
As you advance, you’ll focus on exception handling to manage errors effectively and ensure reliable applications. The course concludes with the application of Java fundamentals in industry-level projects, where you’ll implement the concepts learned throughout the course to solve real-world problems. By the end of the course, you’ll be equipped with the skills to build scalable, efficient, and robust Java applications for professional environments.
OOPS with Java Syllabus
Module 1: Introduction to Java Programming
- Understanding Java syntax, structure, and basic programming concepts
- Setting up the Java development environment (IDE setup, compiling and running Java programs)
- Introduction to Java data types, variables, and operators
Module 2: Control Flow and Decision Making
- Implementing decision-making constructs: if-else, switch-case
- Loops: for, while, do-while for iteration
- Using control flow to build interactive and dynamic programs
Module 3: Bitwise Operators and Advanced Java Concepts
- Introduction to bitwise operators in Java
- Working with operators: AND, OR, XOR, shifts, and complements
- Practical applications of bitwise operators in Java programming
Module 4: Arrays and Data Structures
- Understanding arrays: declaration, initialization, and operations
- Multi-dimensional arrays and array manipulation techniques
- Introduction to basic data structures like lists and arrays
Module 5: Object-Oriented Programming (OOP) Concepts
- Core OOP principles: Encapsulation, Inheritance, Polymorphism, and Abstraction
- Designing Java classes and objects
- Creating reusable code with classes and methods
Module 6: Functions and Methods in Java
- Defining and calling functions in Java
- Passing parameters and returning values from methods
- Method overloading and recursion in Java
Module 7: Condition Handling and Error Management
- Understanding exceptions and how to handle errors in Java
- Using try-catch blocks for exception handling
- Best practices for managing and debugging errors
Module 8: Menu-Driven Programs and Interactive Applications
- Building interactive menu-driven programs using Java
- Implementing user input and controlling application flow based on choices
- Enhancing program usability with menus and options
Module 9: Pattern Printing and Advanced Loops
- Developing pattern printing programs with loops
- Exploring nested loops and their applications
- Designing complex patterns and working with different loop combinations
Module 10: Strings and String Manipulation
- Understanding Java String class and methods
- String concatenation, comparison, and manipulation techniques
- Working with string formatting, parsing, and substring extraction
Object Oriented Programming Projects
Project 1:Student Management System
- Create a system to manage student details, course enrollments, and performance.
- Implement classes for students and courses, using inheritance and polymorphism to track grades and generate reports.
Project 2: Online Banking System
- Build a banking system for account creation, deposits, withdrawals, and transfers.
- Use classes for managing accounts and transactions, applying inheritance to create specialized account types.
Project 3: E-commerce Shopping Cart System
- Develop a shopping cart system to manage product listings, cart operations, and order processing.
- Apply OOP concepts like inheritance and polymorphism for product categories, and encapsulate cart and order management features.
What Students Say...
Certifications
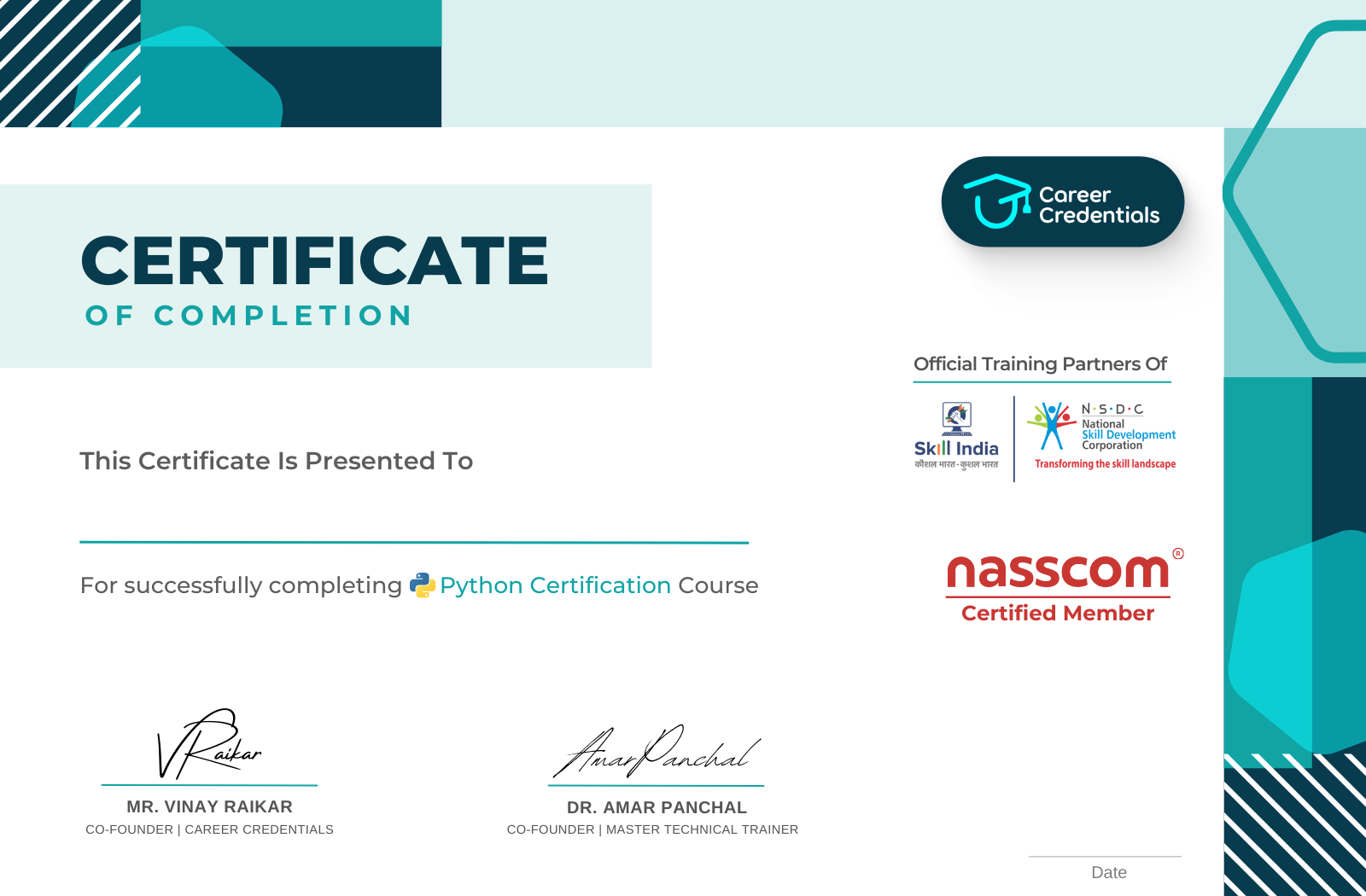
Career Credentials
Python Certified

NSDC
National Certification
- Level
Begineer
- Total Enrolled
449
- Duration
3 Months
- Mode
Online
A course by
Material Includes
- Course Syllabus and Video Lectures: Comprehensive course outline and recorded sessions by Dr. Amar Panchal.
- Hands-On Project Kits: Resources and templates for the 3 industry-level projects, covering web development, data analysis, and automation.
Prerequisites
- Basic understanding of programming concepts.
- A computer with internet access for coding and online resources.
- Willingness to learn and apply agile development practices.
Get Expert Career Guidance for Free!!!
Join us on Free 1:1 Counselling session where we provide personalised Career Guidance for Every Student