Applied Python Programming: A Project-Driven Approach
What Will You Learn?
This course offers a hands-on approach to mastering Python through real-world projects. You’ll dive into essential programming concepts, object-oriented principles, and data handling, building a strong foundation for Python development as you apply your knowledge in practical scenarios.
In addition to core lessons, the course includes three in-depth, industry-level projects designed to reinforce your skills. Under the guidance of Prashant Jha sir, you’ll work on projects in critical areas like data analysis and automation, mimicking real-world tasks encountered in industry roles. This blend of structured lessons and practical application ensures you gain both comprehensive Python knowledge and the real-world experience necessary for career readiness.
Course Flow
The Applied Python Programming: A Project-Driven Approach course begins by building core Python skills. You’ll cover essential programming elements, including syntax, variables, control flow, loops, and an introduction to object-oriented programming. These fundamentals provide a strong foundation in writing and understanding Python code.
From there, the course moves into data handling, exploring the use of lists, dictionaries, and file operations. Additionally, you’ll gain practical skills in error-handling and debugging techniques, essential for efficient and effective coding.
To solidify these concepts, you’ll apply what you’ve learned through three hands-on projects, guided by Prashant Jha sir. These projects cover areas like data analysis and automation, offering a real-world context to your Python skills. This combination of foundational knowledge and project-based learning ensures both technical proficiency and readiness for practical applications.
Applied Python Programming Syllabus
Module 1: Introduction to Python Programming
- Introduction to Python: What is Python and why use it?
- Program structure in Python: Understanding scripts and modules.
- Execution steps: Running Python code interactively and in scripts.
- Interactive Shell: Using the Python interpreter for exploration.
- Memory management: How Python handles data and garbage collection works.
- Dos and Don'ts: Best practices for writing clean and efficient Python code.
Module 2: Operators, Conditional Statements, and Loops
- Assignments, Expressions, and prints: Working with data and output.
- If statements and syntax rules: Making decisions in your code.
- While and For loops: Iterating over sequences and controlling program flow.
- Iterations and comprehensions: Advanced techniques for looping.
Module 3: Data Structures
- Numbers: Working with integers, floats, and complex numbers.
- Strings: Manipulating text data in Python.
- Lists: Creating, accessing, and modifying ordered collections of items.
- Tuples: Immutable ordered sequences for specific use cases.
- Dictionaries: Key-value pairs for storing and organizing data.
Module 4: Functions in Python
- Function definition and call: Creating and using reusable blocks of code.
- Function scope: Understanding variable visibility within functions.
- Arguments: Passing data to and returning data from functions.
Module 5: Object Oriented Programming (OOPS) using Python
- Classes and instances: Creating blueprints for objects.
- Class method calls: Defining behavior for objects.
- Inheritance and composition: Building relationships between classes.
- Polymorphism: Making code adaptable to different object types.
Module 6: Exception Handling in Python Programming
- Default exception handler: Default behavior when errors occur.
- Catching exceptions: Handling specific errors gracefully.
- Raising exceptions: Signaling errors to other parts of your code.
- User-defined exceptions: Creating custom error types for specific scenarios.
Module 7: Python Programming Questions
- Check If a Character is a Vowel or Consonant
- Find the Max and Min Elements in a List
- Add an Element to the Beginning of a List
- Count Vowels and Consonants in a String
- Find the Sum of All Elements in a List
- Calculate the Factorial of a Number
- Even Numbers from 1 to 50
- Reverse a String Using a Loop
- Determine if a Number is +Ve or -Ve or Zero
- Separate +Ve and -Ve Numbers from a List
- Find the Second Largest Element in a List
- Remove Duplicates from a List
- Move an Element to the End of a List
- Calculate the Product of Array Elements Except Itself
Project Module 1: Introduction to Python Fundamentals
- Project: MCQ Assessment Project
- Topics: Variables, loops, conditionals, functions, error handling
- Learning Outcome: Solidify understanding of Python syntax and foundational concepts through hands-on practice.
Project Module 2: Working with Data and File Operations
- Project: Student Data Management System
- Topics: Lists, dictionaries, file handling (TEXT, CSV, Binary), basic data processing
- Learning Outcome: Gain skills in managing and storing data, using Python to automate file- based tasks.
Project Module 3: Object-Oriented Programming (OOP) in Python
- Project: Hotel Booking System
- Topics: Classes, inheritance, encapsulation, polymorphism
- Learning Outcome: Develop modular, reusable code structures, and understand OOP principles to improve code readability and maintainability.
Assignment
- This course will include a combination of assignments, quizzes, and a final project to assess your understanding of the covered concepts.
Applied Python Programming Projects
Project 1: Multiple-Choice Quiz Assessment System
- A set of preloaded multiple-choice questions and options.
- Random question selection or predefined order.
- User input to select an answer.
- Scoring system to track correct and incorrect answers.
- Final score displayed at the end of the quiz.
Project 2: Bank Account Management System
- Account Creation: Users can create different types of accounts (Savings, Current).
- Deposit: Users can deposit money into their accounts.
- Withdrawal: Users can withdraw money, provided they have enough balance.
- Balance Inquiry: Users can check the balance of their account.
Project 3: Hotel Booking System
- Room Booking: Users can book different types of rooms (Standard, Deluxe).
- Check Availability: Users can check the availability of rooms before making a booking.
- Booking Management: Users can view and manage their bookings.
- Room Types: Different room types have different rates and amenities.
What Students Say...
Certifications
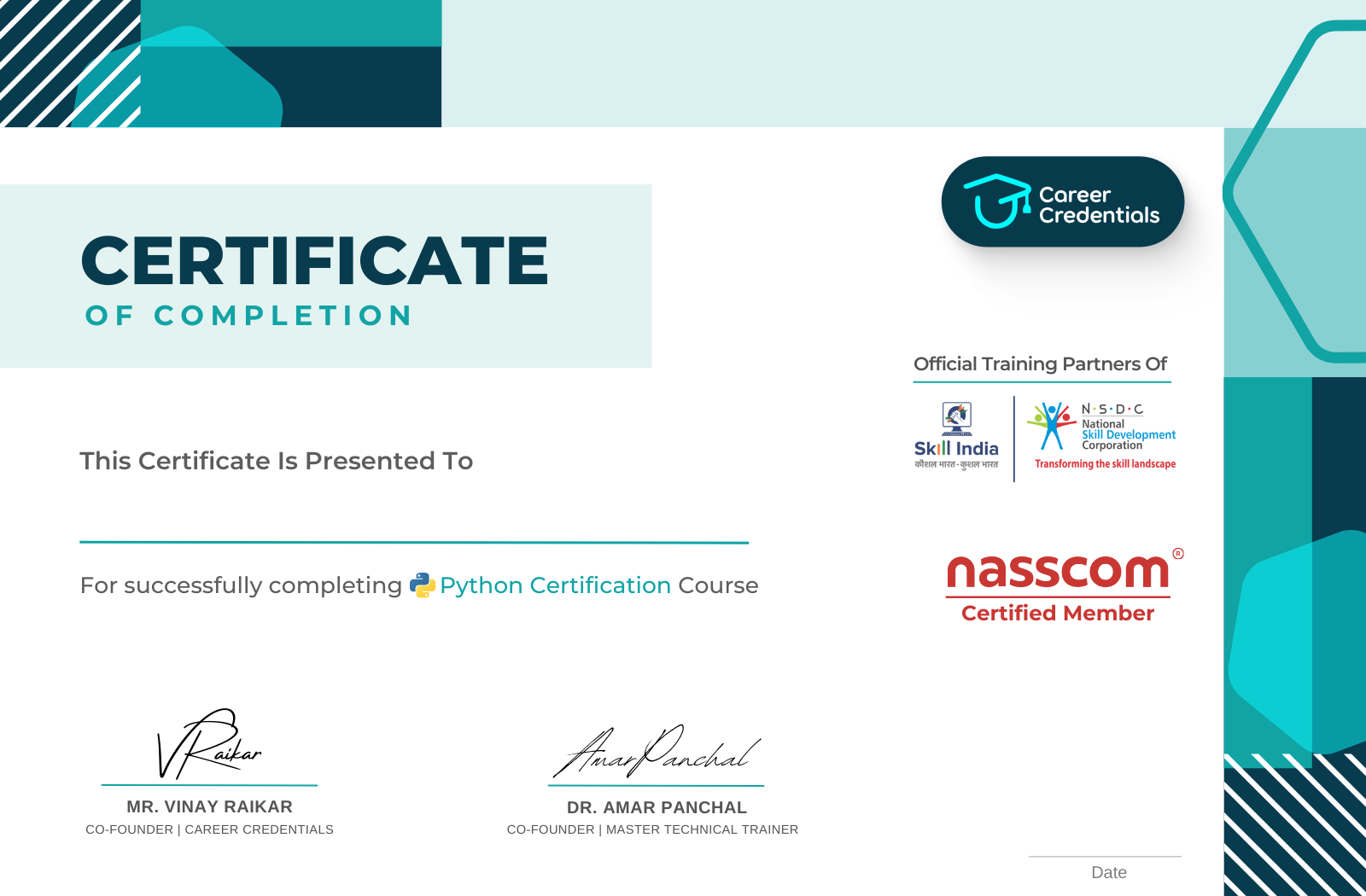
Career Credentials
Python Certified

NSDC
National Certification
- Level
Begineer
- Total Enrolled
329
- Duration
40 Hrs
- Mode
Online
A course by
Material Includes
- Course Syllabus and Video Lectures: Comprehensive course outline and recorded sessions by Prashant Jha sir
- Hands-On Project Kits: Resources and templates for the 3 industry-level projects, covering data analysis, and automation.
- Assignments and Quizzes: Self-paced assignments with solution sets and interactive quizzes to reinforce learning.
Prerequisites
- Basic understanding of programming concepts.
- A computer with internet access for coding and online resources.
- Willingness to learn and apply agile development practices.
Get Expert Career Guidance for Free!!!
Join us on Free 1:1 Counselling session where we provide personalised Career Guidance for Every Student