Starting your coding career with Python
What Will You Learn?
In this Python course, you’ll dive deeply into Python’s core concepts, from foundational syntax and control structures to more advanced areas such as object-oriented programming (OOPs), functions, data structures, and file handling. You’ll become proficient in using loops and conditional statements to create efficient code, tackle error handling to enhance your programming toolkit.
This course also provides extensive practical experience with 24 industry-level projects designed to solidify your skills. Led by Dr. Amar Panchal, you’ll complete 12 diverse projects across key areas like web development, data analysis, and automation, simulating real-world tasks you’ll face in industry roles. Additionally, you’ll receive 12 self-paced assignments aligned with these sessions, enabling you to reinforce concepts and independently tackle projects similar to those previously taught. This dual approach of structured lessons and practical application ensures you’re equipped with the in-depth Python knowledge and real-world experience needed for career readiness.
Course Flow
The Python Fundamentals and Industry-Level Projects course starts with core Python skills, covering syntax, variables, control flow, loops, and object-oriented programming. You’ll move on to data handling with lists, dictionaries, and file operations, along with essential error-handling and debugging techniques.
Next, you’ll apply these skills through 24 practical projects: 12 guided projects with Dr. Amar Panchal in areas like data analysis and automation, followed by 12 self-paced assignments for independent practice. This blend of foundational learning and hands-on application ensures both technical proficiency and real-world readiness.
Level 1 Course Syllabus
Module 1: Introduction to Python Programming
- Introduction to Python: What is Python and why use it?
- Program structure in Python: Understanding scripts and modules.
- Execution steps: Running Python code interactively and in scripts.
- Interactive Shell: Using the Python interpreter for exploration.
- Memory management: How Python handles data and garbage collection works.
- Dos and Don'ts: Best practices for writing clean and efficient Python code.
Module 2: Operators, Conditional Statements, and Loops
- Assignments, Expressions, and prints: Working with data and output.
- If statements and syntax rules: Making decisions in your code.
- While and For loops: Iterating over sequences and controlling program flow.
- Iterations and comprehensions: Advanced techniques for looping.
Module 3: Data Structures
- Numbers: Working with integers, floats, and complex numbers.
- Strings: Manipulating text data in Python.
- Lists: Creating, accessing, and modifying ordered collections of items.
- Tuples: Immutable ordered sequences for specific use cases.
- Dictionaries: Key-value pairs for storing and organizing data.
Module 4: Functions in Python
- Function definition and call: Creating and using reusable blocks of code.
- Function scope: Understanding variable visibility within functions.
- Arguments: Passing data to and returning data from functions.
Module 5: Object Oriented Programming (OOPS) using Python
- Classes and instances: Creating blueprints for objects.
- Class method calls: Defining behavior for objects.
- Inheritance and composition: Building relationships between classes.
- Polymorphism: Making code adaptable to different object types.
Module 6: Exception Handling in Python Programming
- Default exception handler: Default behavior when errors occur.
- Catching exceptions: Handling specific errors gracefully.
- Raising exceptions: Signaling errors to other parts of your code.
- User-defined exceptions: Creating custom error types for specific scenarios.
Module 7: Python Programming Questions
- Check If a Character is a Vowel or Consonant
- Find the Max and Min Elements in a List
- Add an Element to the Beginning of a List
- Count Vowels and Consonants in a String
- Find the Sum of All Elements in a List
- Calculate the Factorial of a Number
- Even Numbers from 1 to 50
- Reverse a String Using a Loop
- Determine if a Number is +Ve or -Ve or Zero
- Separate +Ve and -Ve Numbers from a List
- Find the Second Largest Element in a List
- Remove Duplicates from a List
- Move an Element to the End of a List
- Calculate the Product of Array Elements Except Itself
Assignment
- This course will include a combination of assignments, quizzes, and a final project to assess your understanding of the covered concepts.
Level 2 Course Syllabus
Project 1: To-Do List (Menu-driven, Functions, Basic Data Structures)
- Users can add, remove, or edit tasks via a menu-driven interface, providing an intuitive user experience.
- Functions are utilized to organize the code effectively, improving readability and maintainability.
- Basic data structures, such as lists, are used to store and manage tasks, introducing practical data handling and manipulation within Python.
Student Project: Contact Management System
- Manage contacts efficiently with CRUD operations (Create, Read, Update, Delete) to organize personal or professional contact information.
Project 2: Billing System (Loops, Conditionals, Calculations)
- Simulates a billing system for placing orders, allowing users to manage and process transactions.
- Loops iterate through ordered items to ensure accurate billing.
- Conditional statements calculate total cost based on quantity and price.
- Provides practical experience with financial computations in Python through basic calculations for bill generation.
Student Project: Library Fine Calculator
- Calculate overdue library fines based on return dates, providing practical experience with conditional logic.
Project 3: Banking System (Object-Oriented Programming - OOP)
- Manages user accounts through classes and objects, implementing OOP principles.
- Features include user registration, deposits, withdrawals, and balance checks.
- Includes error handling for negative values and ensures a minimum balance is maintained, demonstrating practical applications of OOP in banking scenarios.
Student Project: Library Management System
- A basic management system for handling library book records, focusing on data organization and basic CRUD operations.
Project 4: Translator (Modules)
- Utilizes the translate module to convert text between languages.
- Offers a menu-driven interface for selecting source and target languages and entering text.
- Showcases the use of external modules to expand Python’s functionality for language processing.
Student Project: Personalized Poem Generator
- Generates custom poems based on user input, using string manipulation techniques for creative output.
Project 5: Sketch Maker (Modules)
- Takes an image as input and uses an image processing library to create a sketch version.
- Demonstrates Python’s capability to work with external libraries, achieving complex image transformations.
Student Project: OCR Text Extraction from Documents
- Extracts text from images or scanned documents using OCR libraries, demonstrating Python’s capability with external modules.
Project 6: ChatGPT/Gemini Communication (Modules)
- Explores Python’s ability to interact with large language models like ChatGPT or Gemini through APIs.
- Demonstrates connecting to these models to send and receive text data, showcasing API integration for advanced AI management.
- Additional research on API access and communication specifics may be needed.
Student Project: Weather Bot
- Fetches weather information based on user input, showcasing API integration for real-time data retrieval.
Project 7: Letter Generator (GUI, String Manipulation, Files)
- Accepts user details (e.g., name, date, content) and generates a formatted letter through string manipulation.
- Saves the generated letter to a file, with optional GUI implementation (e.g., Tkinter) to improve user experience.
Student Project: International E-Commerce Language Translation
- Implements language translation in an e-commerce setting, enabling users to view content in different languages.
Project 8: Interactive Game (Console-based)
- Creates a text-based game with user interaction and decision-making elements.
- Introduces random numbers to add variability, making the gameplay more engaging by affecting outcomes based on user choices.
Student Project: Interactive Game
- A console-based game where players guess a number, using loops and conditionals to create an engaging user experience.
Project 9: Data Analyzer App (GUI, Modules)
- Designed for data analysis, using a specific data analysis library to process and interpret information.
- Incorporates basic GUI elements for user interaction and data visualization, highlighting Python’s use in effective data manipulation.
Student Project: Sales Data Analysis
- Analyzes sales data using Python libraries, providing insights into data manipulation and visualization.
Project 10: My Secret Writer (GUI, Files, Encryption)
- Enables users to write and encrypt secret messages using basic symmetric encryption techniques.
- Uses file handling to securely store encrypted messages, with an optional GUI for a user-friendly experience in encryption and decryption.
Student Project: GUI Application for Encrypted Messaging
- Allows users to send encrypted messages securely, utilizing GUI elements for an intuitive interface.
Project 11: Contact App (CRUD - Create, Read, Update, Delete)
- Manages contacts efficiently through CRUD operations using a database library like PyDB.
- Allows users to add, remove, search, and edit contact information, offering a complete solution for managing contacts.
Student Project: Task Management Application
- Manage tasks effectively with options to add, update, and delete tasks, showcasing basic data handling skills.
Project 12: Inventory Management (Data Structures, Automation)
- Simulates an inventory management system, handling stock data through dictionaries or lists.
- Includes order handling and may feature auto-reordering based on inventory levels, potentially using automation libraries for streamlined stock management.
Student Project: Student Project: Rental Management System
- A system for tracking rental items and dates, focusing on data structures and basic automation techniques for inventory management.
What Students Say...
Certifications
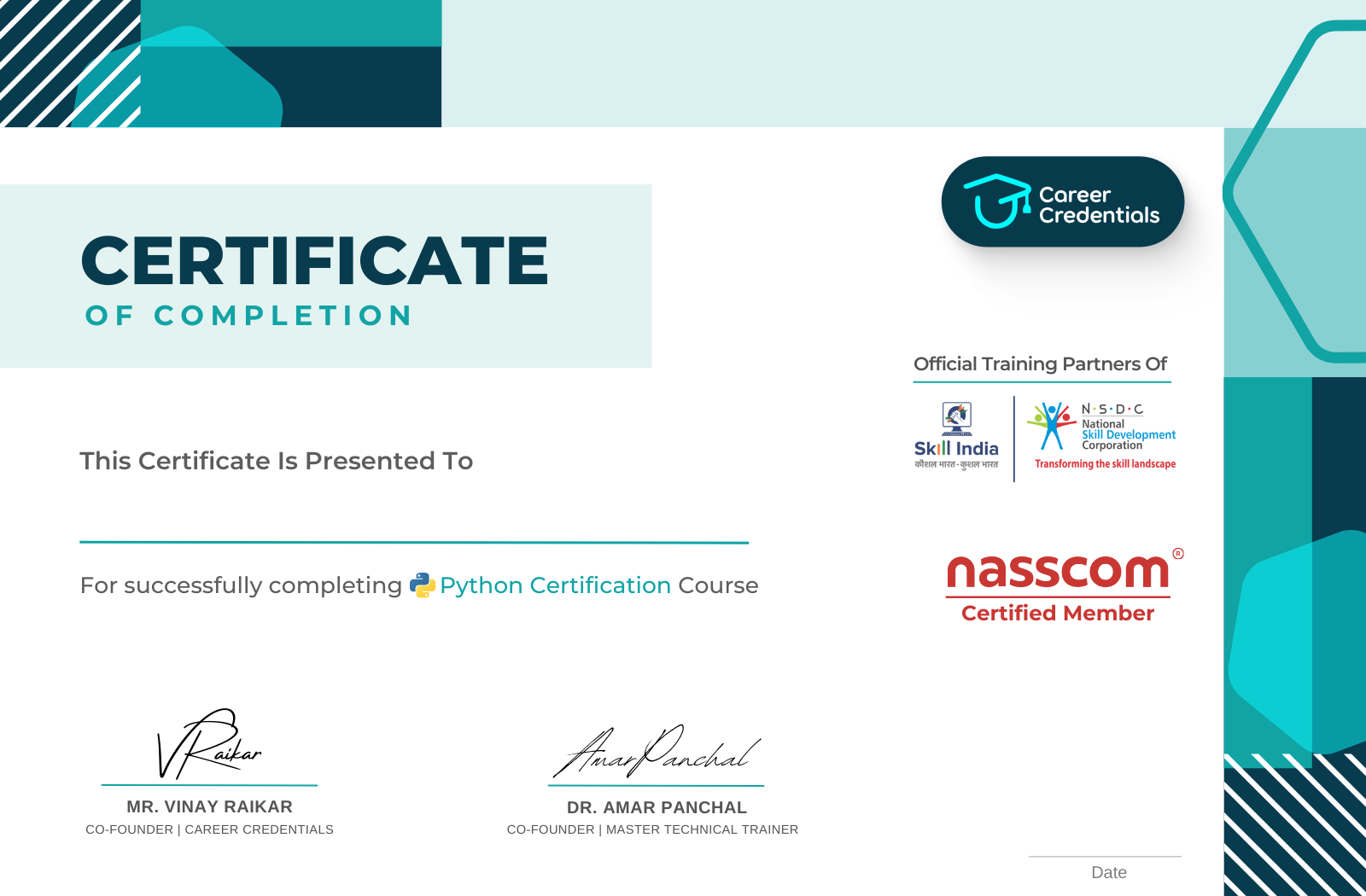
Career Credentials
Python Certified

NSDC
National Certification
- Price
₹ 10000
- Level
Intermediate
- Total Enrolled
554
- Duration
2 Months
- Mode
Online
A course by
Material Includes
- Course Syllabus and Video Lectures: Comprehensive course outline and recorded sessions by Dr. Amar Panchal.
- Hands-On Project Kits: Resources and templates for the 24 industry-level projects, covering web development, data analysis, and automation.
- Assignments and Quizzes: Self-paced assignments with solution sets and interactive quizzes to reinforce learning.
Prerequisites
- Basic understanding of programming concepts.
- A computer with internet access for coding and online resources.
- Willingness to learn and apply agile development practices.
Get Expert Career Guidance for Free!!!
Join us on Free 1:1 Counselling session where we provide personalised Career Guidance for Every Student